10 Essential React Interview Questions For Aspiring Frontend Developers
10 Essential React Interview Questions For Aspiring Frontend Developers
Comprehensive React Cheatsheet included at the bottom of this article!
Resources:
All of the code examples below will be included a second time at the bottom of this article as an embedded gist.javascript.plainenglish.io
As I learn to build web applications in React I will blog about it in this series in an attempt to capture the…bryanguner.medium.com
A JavaScript library for building user interfaces Declarative React makes it painless to create interactive UIs. Design…github.com
Also … here is my brand new blog site… built with react and a static site generator called GatsbyJS!
It’s a work in progress
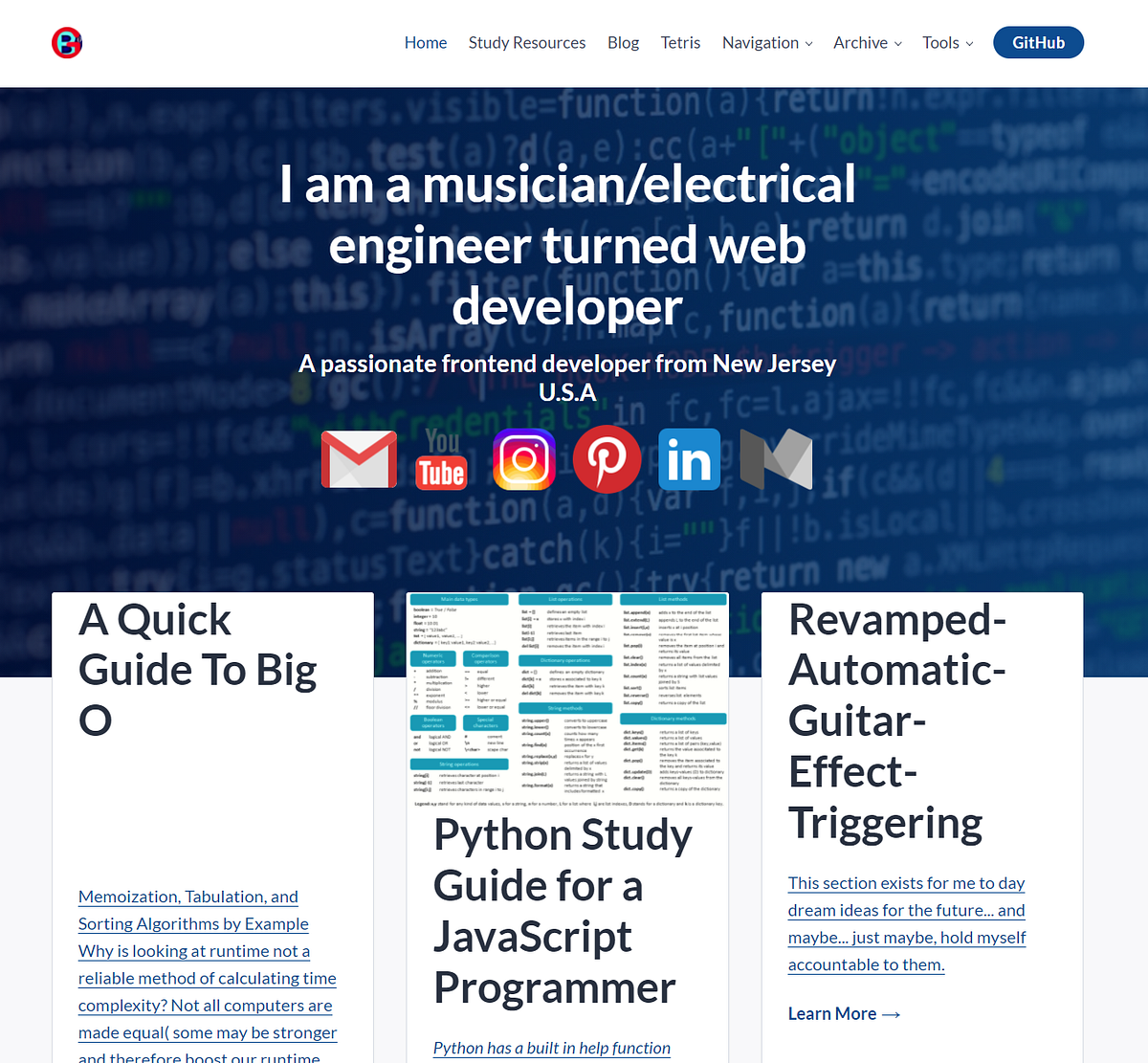
Beginning of the Article:
Pros
- Easy to learn
- HTML-like syntax allows templating and highly detailed documentation
- Supports server-side rendering
- Easy migrating between different versions of React
- Uses JavaScript rather than framework-specific code
Cons
- Poor documentation
- Limited to only view part of MVC
- New developers might see JSC as a barrier
Where to Use React
- For apps that have multiple events
- When your app development team excels in CSS, JavaScript and HTML
- You want to create sharable components on your app
- When you need a personalized app solution
Misconceptions about React
React is a framework:
Many developers and aspiring students misinterpret React to be a fully functional framework. It is because we often compare React with major frameworks such as Angular and Ember. This comparison is not to compare the best frameworks but to focus on the differences and similarities of React and Angular’s approach that makes their offerings worth studying. Angular works on the MVC model to support the Model, View, and Controller layers of an app. React focuses only on the ‘V,’ which is the view layer of an application and how to make handling it easier to integrate smoothly into a project.
React’s Virtual DOM is faster than DOM.
React uses a Virtual DOM, which is essentially a tree of JavaScript objects representing the actual browser DOM. The advantage of using this for the developers is that they don’t manipulate the DOM directly as developers do with jQuery when they write React apps. Instead, they would tell React how they want the DOM to make changes to the state object and allow React to make the necessary updates to the browser DOM. This helps create a comprehensive development model for developers as they don’t need to track all DOM changes. They can modify the state object, and React would use its algorithms to understand what part of UI changed compared to the previous DOM. Using this information updates the actual browser DOM. Virtual DOM provides an excellent API for creating UI and minimizes the update count to be made on the browser DOM.
However, it is not faster than the actual DOM. You just read that it needs to pull extra strings to figure out what part of UI needs to be updated before actually performing those updates. Hence, Virtual DOM is beneficial for many things, but it isn’t faster than DOM.
1. Explain how React uses a tree data structure called the virtual DOM to model the DOM
The virtual DOM is a copy of the actual DOM tree. Updates in React are made to the virtual DOM. React uses a diffing algorithm to reconcile the changes and send the to the DOM to commit and paint.
2. Create virtual DOM nodes using JSX To create a React virtual DOM node using JSX, define HTML syntax in a JavaScript file.
Here, the JavaScript hello variable is set to a React virtual DOM h1 element with the text “Hello World!”.
You can also nest virtual DOM nodes in each other just like how you do it in HTML with the real DOM.
3. Use debugging tools to determine when a component is rendering
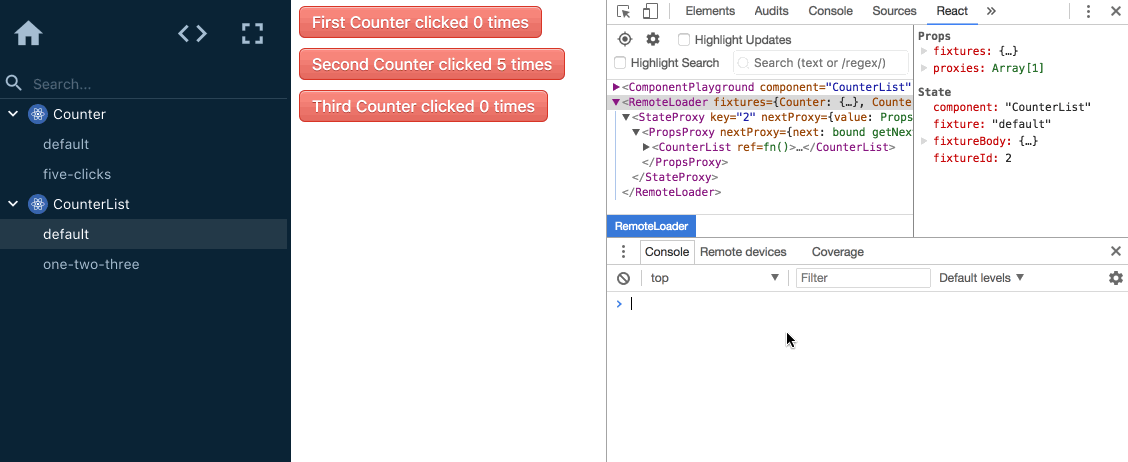
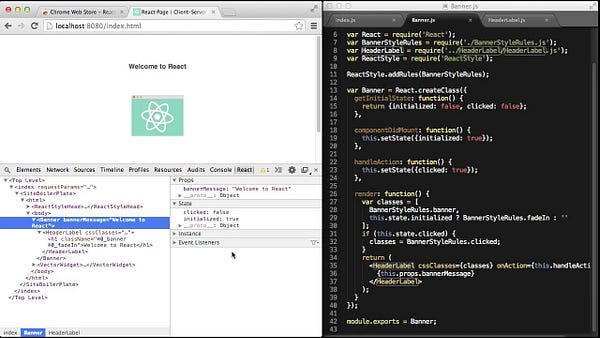
We use the React DevTools extension as an extension in our Browser DevTools to debug and view when a component is rendering
4. Describe how JSX transforms into actual DOM nodes
- To transfer JSX into DOM nodes, we use the ReactDOM.render method. It takes a React virtual DOM node’s changes allows Babel to transpile it and sends the JS changes to commit to the DOM.
5. Use the ReactDOM.render
method to have React render your virtual DOM nodes under an
actual DOM node
6. Attach an event listener to an actual DOM node using a virtual node
The virtual DOM (VDOM) is a programming concept where an ideal, or “virtual”, representation of a UI is kept in memory and synced with the “real” DOM by a library such as ReactDOM. This process is called reconciliation.
This approach enables the declarative API of React: You tell React what state you want the UI to be in, and it makes sure the DOM matches that state. This abstracts out the attribute manipulation, event handling, and manual DOM updating that you would otherwise have to use to build your app.
Since “virtual DOM” is more of a pattern than a specific technology, people sometimes say it to mean different things. In React world, the term “virtual DOM” is usually associated with React elements since they are the objects representing the user interface. React, however, also uses internal objects called “fibers” to hold additional information about the component tree. They may also be considered a part of “virtual DOM” implementation in React.
Is the Shadow DOM the same as the Virtual DOM?
No, they are different. The Shadow DOM is a browser technology designed primarily for scoping variables and CSS in web components. The virtual DOM is a concept implemented by libraries in JavaScript on top of browser APIs.
- To add an event listener to an element, define a method to handle the event and associate that method with the element event you want to listen for:
7. Use create-react-app
to initialize a new React app and import required
dependencies
- Create the default create-react-application by typing in our terminal
Explanation of npm vs npx from Free Code Camp:
npm (node package manager) is the dependency/package manager you get out of the box when you install Node.js. It provides a way for developers to install packages both globally and locally.
Sometimes you might want to take a look at a specific package
and try out some commands. But you cannot do that without
installing the dependencies in your local
node_modules
folder.
npm the package manager
npm is a couple of things. First and foremost, it is an online repository for the publishing of open-source Node.js projects.
Second, it is a CLI tool that aids you to install those packages and manage their versions and dependencies. There are hundreds of thousands of Node.js libraries and applications on npm and many more are added every day.
npm by itself doesn’t run any packages. If you want to run a
package using npm, you must specify that package in your
package.json
file.
When executables are installed via npm packages, npm creates links to them:
-
local
installs have links created at the
./node_modules/.bin/
directory -
global
installs have links created from the global
bin/
directory (for example:/usr/local/bin
on Linux or at%AppData%/npm
on Windows)
To execute a package with npm you either have to type the local path, like this:
$ ./node_modules/.bin/your-package
or you can run a locally installed package by adding it into
your
package.json
file in the scripts section, like this:
{
"name": "your-application",
"version": "1.0.0",
"scripts": {
"your-package": "your-package"
}
}
Then you can run the script using
npm run
:
npm run your-package
You can see that running a package with plain npm requires quite a bit of ceremony.
Fortunately, this is where npx comes in handy.
npx the package runner
Since npm version 5.2.0 npx is pre-bundled with npm. So it’s pretty much a standard nowadays.
npx is also a CLI tool whose purpose is to make it easy to install and manage dependencies hosted in the npm registry.
It’s now very easy to run any sort of Node.js-based executable that you would normally install via npm.
You can run the following command to see if it is already installed for your current npm version:
$ which npx
If it’s not, you can install it like this:
$ npm install -g npx
Once you make sure you have it installed, let’s see a few of the use cases that make npx extremely helpful.
Run a locally installed package easily
If you wish to execute a locally installed package, all you need to do is type:
$ npx your-package
npx will check whether
<command>
or
<package>
exists in
$PATH
, or in
the local project binaries, and if so it will execute it.
Execute packages that are not previously installed
Another major advantage is the ability to execute a package that wasn’t previously installed.
Sometimes you just want to use some CLI tools but you don’t want to install them globally just to test them out. This means you can save some disk space and simply run them only when you need them. This also means your global variables will be less polluted.
Now, where were we?
npx create-react-app <name of app> --use-npm
-
npx gives us the latest version.
--use-npm
just means to use npm instead of yarn or some other package manager
8. Pass props into a React component
-
props
is an object that gets passed down from the parent component to the child component. The values can be of any data structure including a function (which is an object)
- You can also interpolate values into JSX.
- Set a variable to the string, “world”, and replace the string of “world” in the NavLinks JSX element with the variable wrapped in curly braces:
Accessing props:
To access our props object in another component we pass it the props argument and React will invoke the functional component with the props object.
Reminder:
Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen.
Function and Class Components
The simplest way to define a component is to write a JavaScript function:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
This function is a valid React component because it accepts a single “props” (which stands for properties) object argument with data and returns a React element. We call such components “function components” because they are literally JavaScript functions.
You can also use an ES6 class to define a component:
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
The above two components are equivalent from React’s point of view.
- You can pass down as many props keys as you want.
9. Destructure props
You can destructure the props object in the function component’s parameter.
10. Create routes using components from the react-router-dom package
a. Import the react-router-dom package:
npm i react-router-dom
In your index.js:
- Above you import your BrowserRouter with which you can wrap your entire route hierarchy. This makes routing information from React Router available to all its descendent components.
- Then in the component of your choosing, usually top tier such as App.js, you can create your routes using the Route and Switch Components
Discover More:
Memoization, Tabulation, and Sorting Algorithms by Example Why is looking at runtime not a reliable method of…bgoonz-blog.netlify.app
REACT CHEAT SHEET:
More content at plainenglish.io
Comments
Post a Comment
Share your thoughts!